As this ejb and mbean both going to be deployed in Jboss
server I have implemented in a way that both are implemented in same EAR
project, but ejb and mbean is made keeping their differences of implementation.
First Let's implement EJB part.
1.
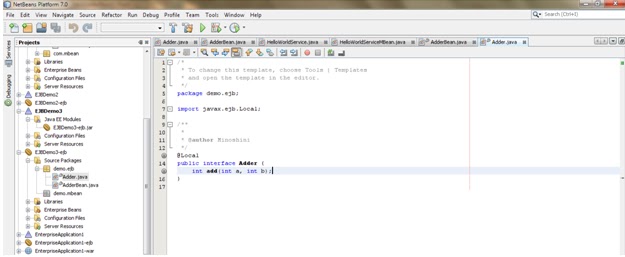 |
Figure 1- Implementation of Adder Interface |
2.
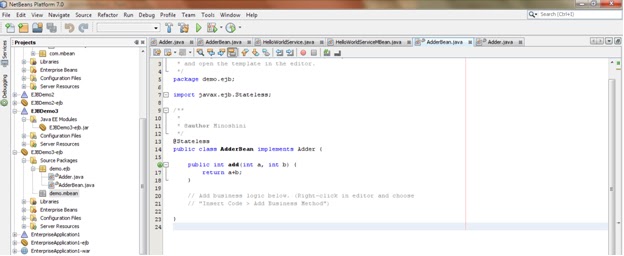 |
Figure 2- Implementation of AdderBean
|
3. Then clean and build the project EJBDemo3
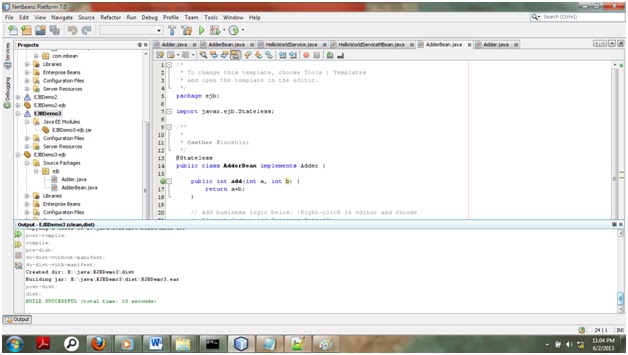 |
Figure 3- building the project EJBDemo3
|
4. Then go to dist folder in EJB and find that EJBDemo3.ear
file and deploy in Jboss server in your working instance. When you deploy it
you will see this kind of output in the command prompt.
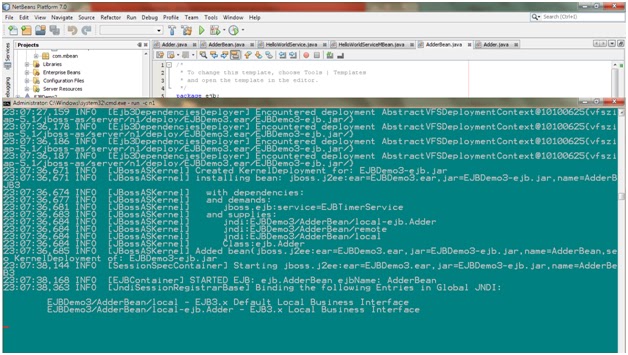 |
Figure 4 - Command prompt view of the output |
Now we are going to
create mbean
5. When creating mbean service there are some jars you should
have to add as libraries. Those are
Jakarta Commons IO,
Jakarta Commons Logging,
jboss-common.jar,
jboss-jmx.jar ,
jboss-system.jar,
jboss-xml-binding.jar
And in this case we need another Jar called org.jboss.annotation.ejb.Service
J
6. AdderService implementation
/*
* To change this
template, choose Tools | Templates
* and open the
template in the editor.
*/
package demo.mbean;
import org.jboss.system.ServiceMBeanSupport;
import javax.naming.InitialContext;
import javax.naming.NamingException;
import javax.rmi.PortableRemoteObject;
import org.jboss.annotation.ejb.Service;
import demo.ejb.Adder;
/**
*
* @author Minoshini
*/
@Service (objectName = "demo.mbean:service=Adder")
public class AdderService extends ServiceMBeanSupport
implements AdderServiceMBean{
private int a=2;
private int b=2;
public int getA() {
return a;
}
public void
setA(int a) {
this.a = a;
}
public int getB()
{
return b;
}
public void
setB(int b) {
this.b = b;
}
public int add(){
try {
InitialContext ctx = new InitialContext();
Object ref
= ctx.lookup("EJBDemo3/AdderBean/local");
Adder adder
= (Adder) PortableRemoteObject.narrow(ref,Adder.class);
return
adder.add(a, b);
} catch
(NamingException e) {
e.printStackTrace();
return 0;
}
}
// The lifecycle
protected void
startService() throws Exception
{
log.info("Starting of AdderService");
}
protected void
stopService() throws Exception
{
log.info("Stopping of AdderService");
}
}
7. AdderServiceMBean
/*
* To change this
template, choose Tools | Templates
* and open the
template in the editor.
*/
package demo.mbean;
/**
*
* @author Minoshini
*/
public interface AdderServiceMBean {
int getA();
void setA(int a);
int getB();
void setB(int b);
public int add();
}
8. After that again clean and build the EJBDemo3 Project
9. Then go the build project like this E:\java\EJBDemo3\EJBDemo3-ejb\build
In the META-INF folder there is a jboss.xml and rename it as jboss-service.xml
and edit it like this.
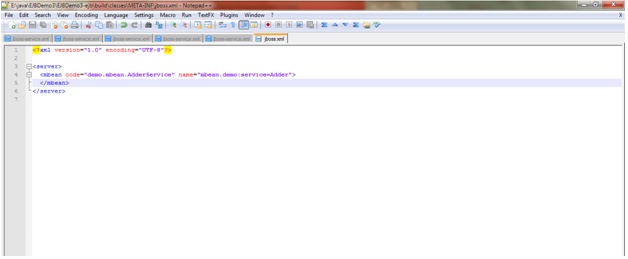 |
Figure 5- jboss-service.xml |
10. Then we are going to create the .SAR folder structure to
deploy in jboss server. It should follow this order.
adder-service.sar
adder-service.sar/META-INF/jboss-server.xml
adder-service.sar/demo/mbean/AdderService.class
adder-service.sar/demo/mbean/AdderServiceMBean.class
11. Then deploy this adder-service.sar folder in deploy folder
of Jboss instance you are working with.
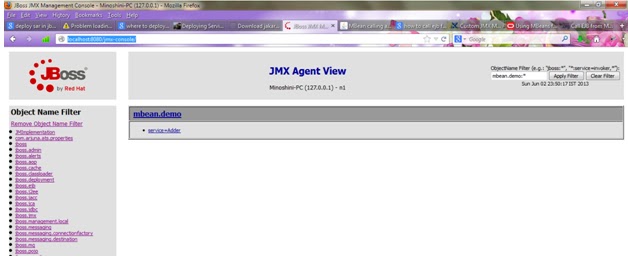 |
Figure 6- Service Adder |
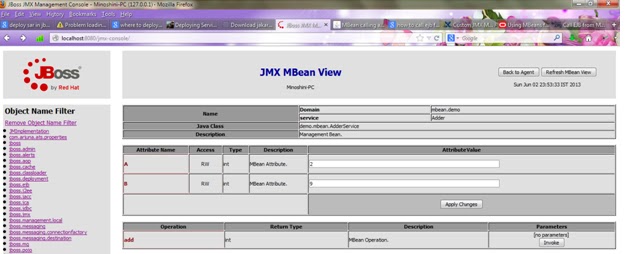 |
Figure 7- JMX MBean view |
From the mbean you can enter inputs also. When you call the
add it will direct to ejb and retur the result.
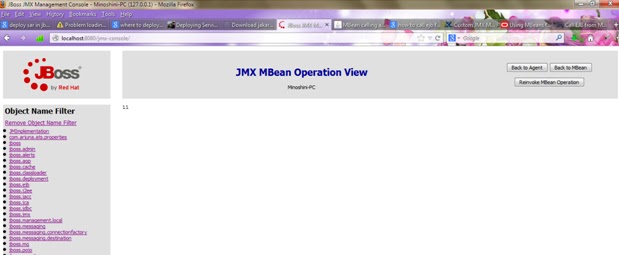 |
Figure 8- Output of the EJB via JMX-Console |